Task 16
Interface and application programming
In this week, I was given a task to have a group assignment and an individual assignment. The group assignment was to compare as many tool options as possible, and the individual assignment was to write an application that interfaces a user with a input &/or output device that you made
Group Task
For this week, we all started with the group task together. Some of us used Processing, Blynk, PyQt5 and others used the Python or Matlab
Blynk
Blynk was designed for the Internet of Things. It can control hardware remotely, it can display sensor data, it can store data, vizualize it and do many other cool things. Blynk supports hardware platforms such as Arduino, Raspberry Pi, and similar microcontroller boards to build hardware for your projects. Blink can support the following Ethernet, Wi-Fi, Bluetooth, Cellular and Serial connection types:
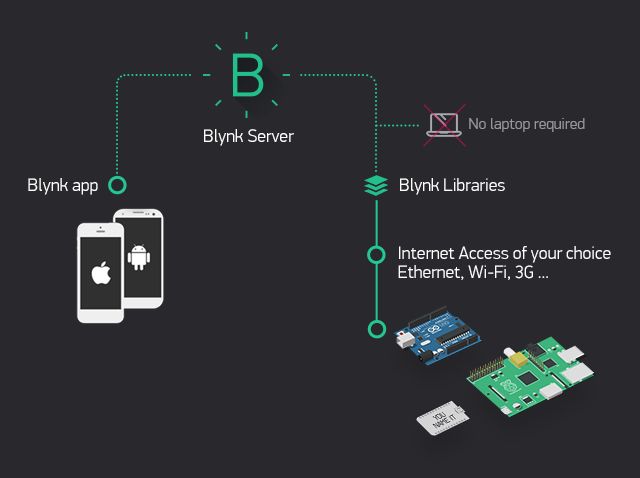
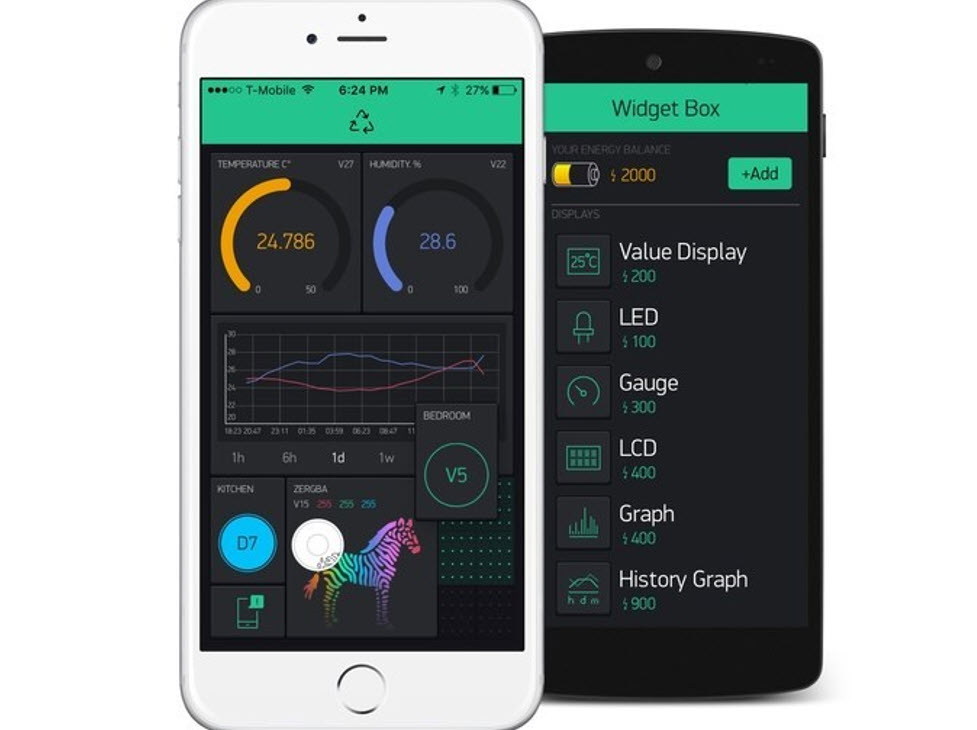
- There are three major components in the platform:
- Blynk App: allows to you create amazing interfaces for your projects using various widgets we provide..
- Blynk Server: responsible for all the communications between the smartphone and hardware. You can use the Blynk Cloud or run your private Blynk server locally. It’s open-source, could easily handle thousands of devices and can even be launched on a Raspberry Pi.
- Blynk Libraries: for all the popular hardware platforms - enable communication with the server and process all the incoming and outcoming commands
- Hello world with Blynk from the microcontroller to Phones Blynk app monitor looks like this:
BLYNK_LOG1("Hello World"); // Print a string
PyQt5
PyQt is a GUI widgets toolkit. It is a Python interface for Qt, one of the most powerful, and popular cross-platform GUI library. PyQt is a blend of Python programming language and the Qt library.Qt is set of cross-platform C++ libraries that implement high-level APIs for accessing many aspects of modern desktop and mobile systems. These include location and positioning services, multimedia, NFC and Bluetooth connectivity, a Chromium based web browser, as well as traditional UI development.
PyQt is used for developing desktop application using python. Latest version is PyQt5 in which we can design window, text box, label, etc using designer application. By default, if we click on the x button on the titlebar, the QWidget is closed. Sometimes we want to modify this default behaviour. For example, if we have a file opened in an editor to which we did some changes. We show a message box to confirm the action. We show a message box with two buttons: Yes and No. The first string appears on the titlebar. The second string is the message text displayed by the dialog. The third argument specifies the combination of buttons appearing in the dialog. The last parameter is the default button. It is the button, which has initially the keyboard focus. The return value is stored in the reply variable.
Below is the message code after running the python code.
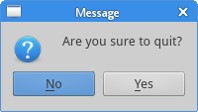
Python
Python is an object-oriented, high-level programming language with integrated dynamic semantics primarily for web and app development. It is extremely attractive in the field of Rapid Application Development because it offers dynamic typing and dynamic binding options. Python is also used for developing complex scientific and numeric applications and its design philosophy emphasises code readability with its notable use of significant whitespace
A simple program for addition and calculating savings in Python is presented below :
# Create a variable savings
savings = 100
# Create a variable factor
factor = 1.10
# Calculate result after 7 years
result = savings * (factor ** 7)
# Print out result
print (result)
The Hello world printed by simply using this:
print(“Hello, World!”)
We followed a this tutorial to control the RGB LED with Python and Arduino.
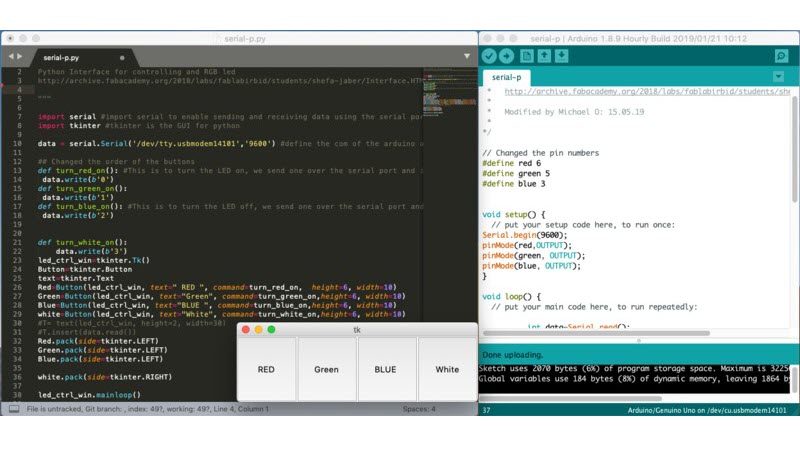
Processing
Processing is a programming language designed for the visual arts community. It is open source and uses basic syntax for creating drawings, animations, and interactive programs. It also includes a basic IDE, which serves as the programming interface.Each program created in Processing is called a "sketch" and can be saved in a sketchbook. I decided to use processing for my Individual Task.
Individual Task
For the Individual Task, I used Processing. It is a software through which we can design a GUI Application and connect them to control the Arduino and other Microcontroller throught serial communication. It supports Javascript language for making the user interface. I utilized the Week 11 input week Ultrasonic sensor for this purpose to acquire sensore data and display it on my GUI Application. Actually before starting with Processing I should have an Arduino code that deals with serial monitor successfuly. Since in Week 11, I did the coding with Atmel Studio 7, thereby I did the coding for the ulrasonic sensor with arduino to acquire serial data in cm. Below is the Arduino Code:
Hardware Setup
The Board was connected to the computer. Below is the board with ultrasonic sensor and the FTDI cable connected to the computer
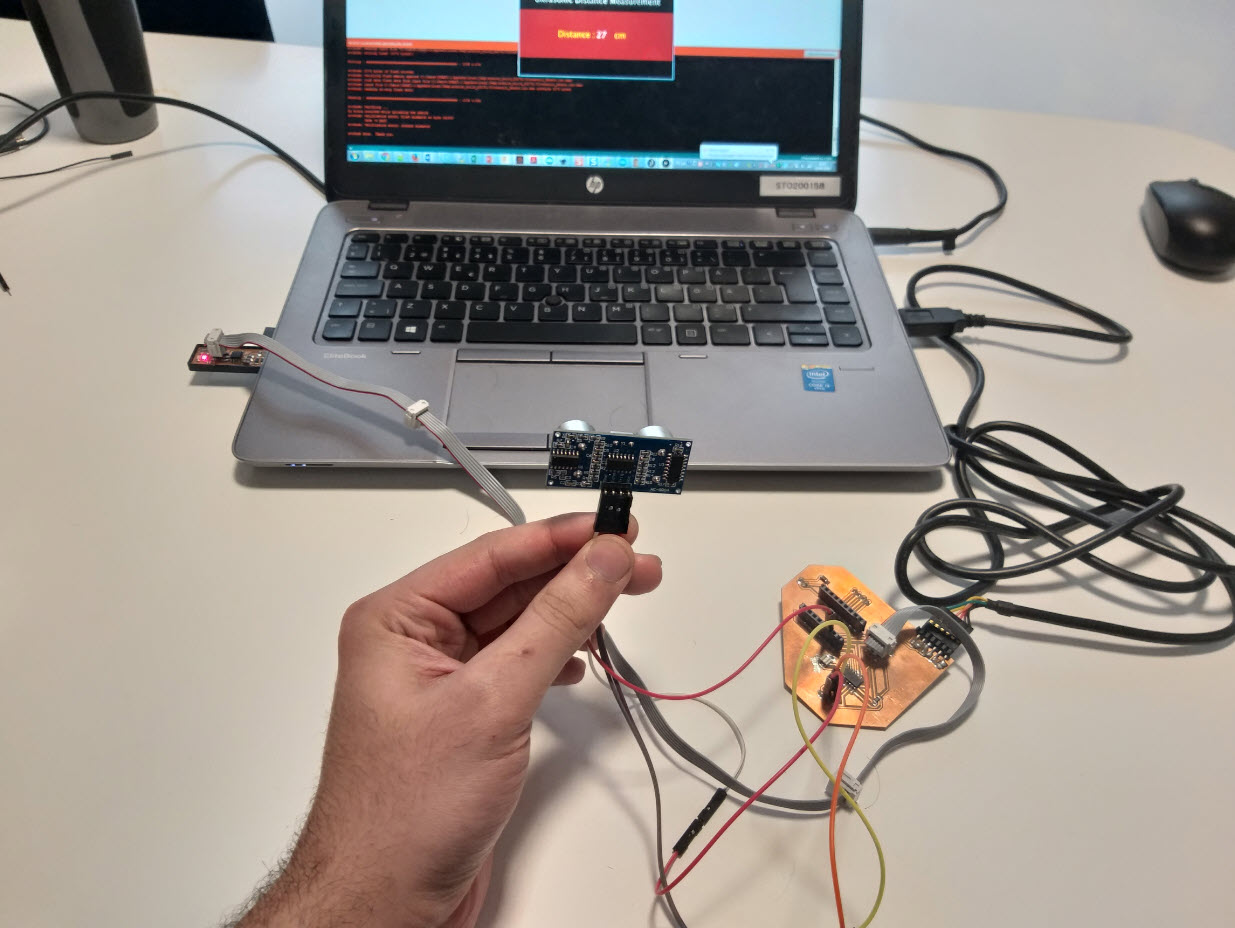
Arduino IDE Code: Ultrasonic Sensor
In the code, the format of the data is distance i.e. string "Serial.println(distance)" with a "\n" that is being received at the receiver serially.
const int trigpin= 8;
const int echopin= 7;
long duration=0;
int distance=0;
void setup()
{
pinMode(trigpin,OUTPUT);
pinMode(echopin,INPUT);
Serial.begin(9600);
}
void loop()
{
digitalWrite(trigpin,HIGH);
delayMicroseconds(10);
digitalWrite(trigpin,LOW);
duration=pulseIn(echopin,HIGH);
distance = duration*0.034/2;
Serial.println(distance);
}
ATTinyCore is used to program ATTiny with Arduino IDE :https://github.com/SpenceKonde/ATTinyCore . From ATTinyCore instructions: “AttinyCore provides a , a built-in software serial named Serial is provided to maximize compatibility, […]. TX is AIN0 (PA1). So no need for Software Serial library needed. That is why Serial is used in the code instead of SoftwareSerial.
To begin with, it was somehow hard for me to deal with this software. Firstly, I opened the sketch file and added the serial library to be able to take serial input from board through serial port
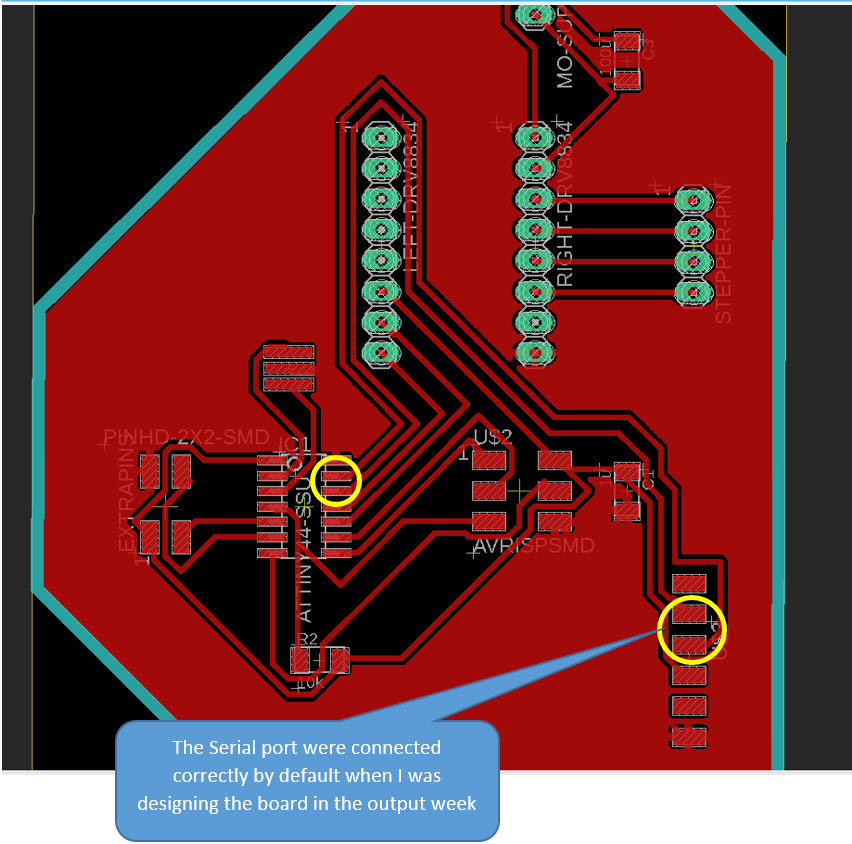
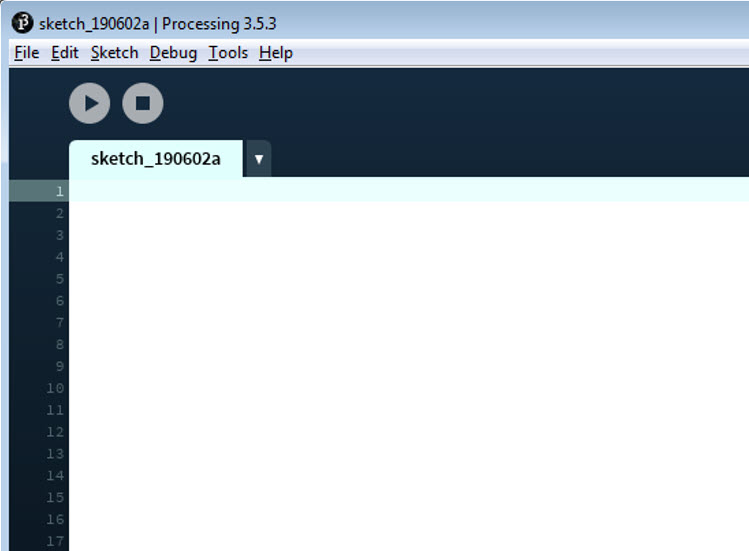
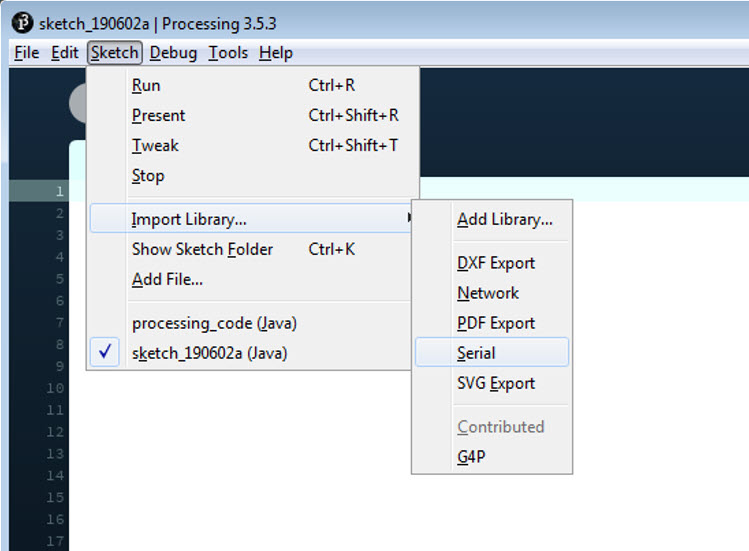
After adding libraries I start playing with simple instructions i.e size and background and see the effect of them such as shown. The Tutorial: 01,Tutorial: 02, Tutorial: 03 and Tutorial: 04 were helpful to get to know about the software commands.I used COM port 3 and baud rate of 9600 for serial communication.
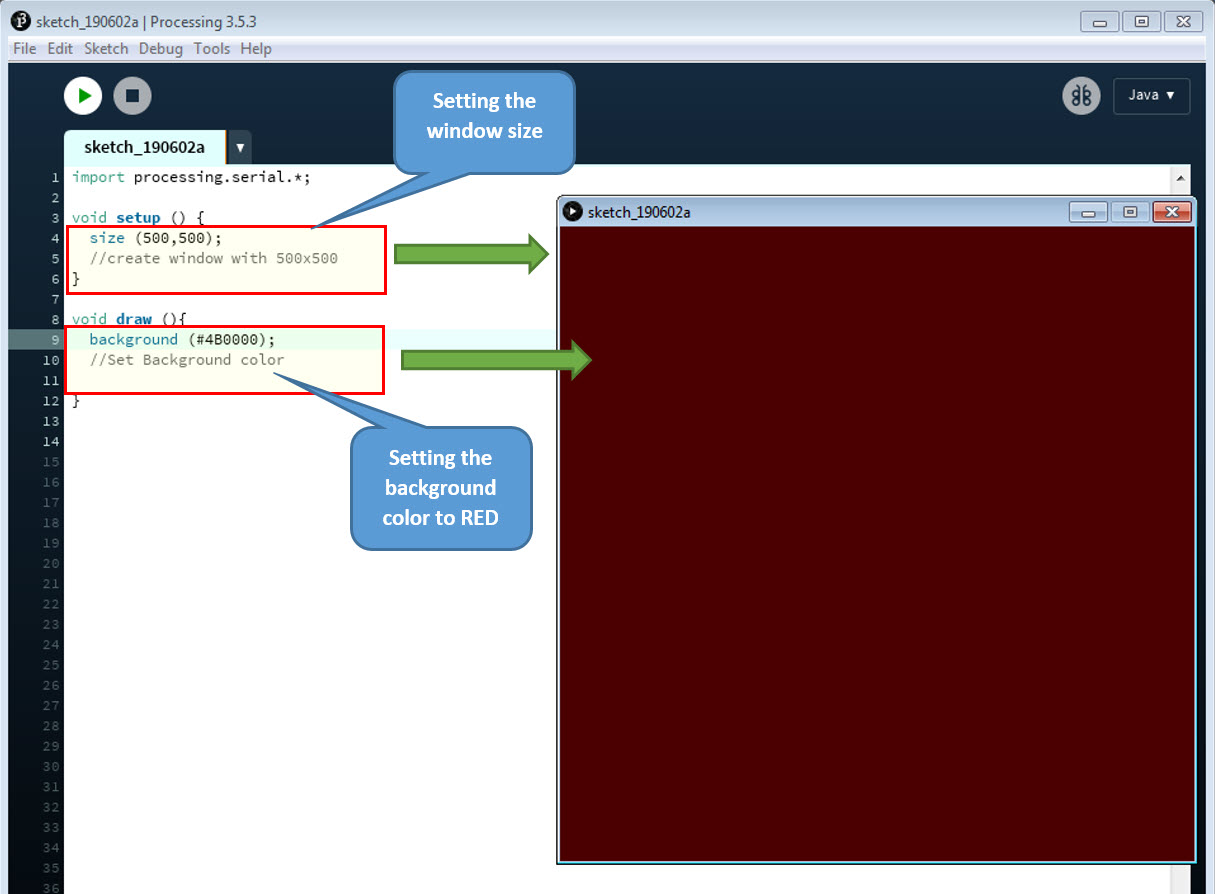
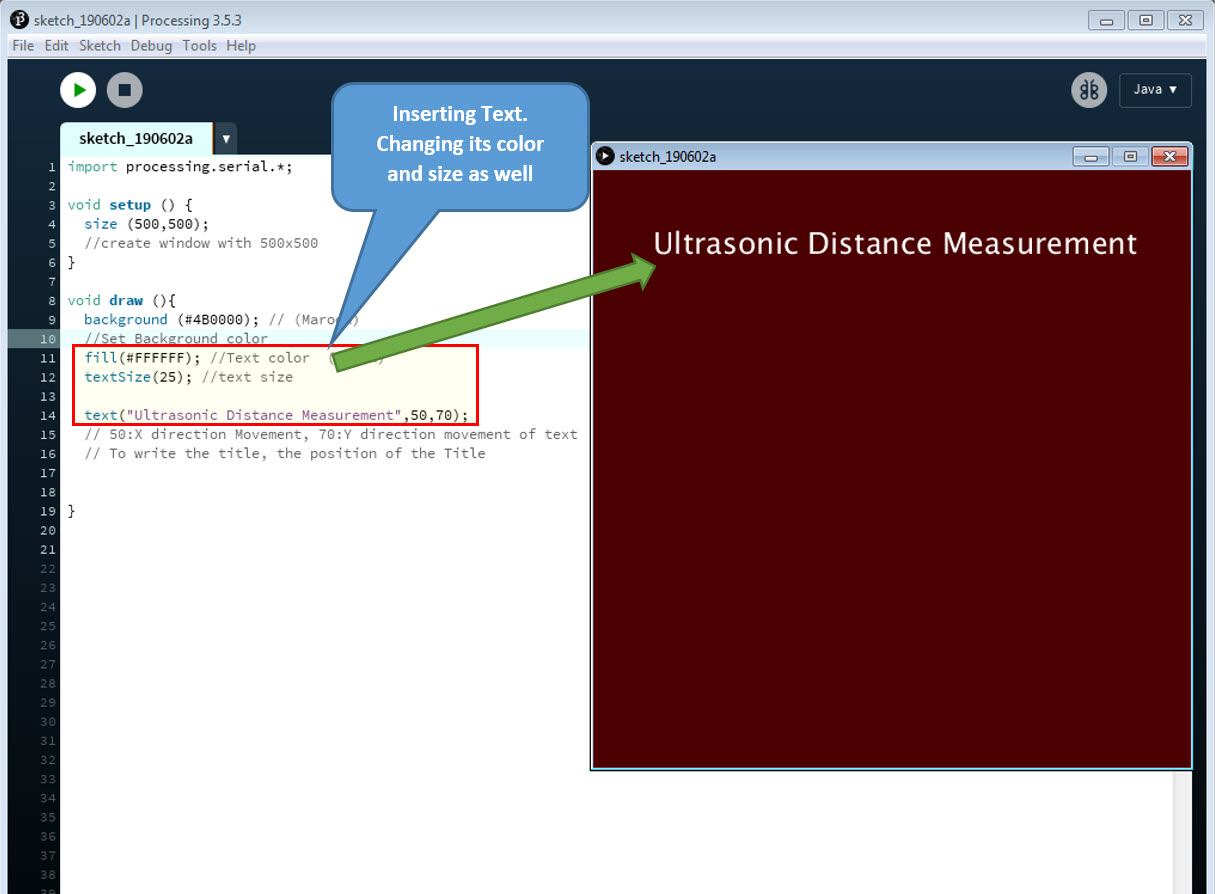
After Couple of trials and errors and following the tutorials mentioned, I was able to get some success with the code and the GUI was able to display the data sent serially from my sensor board. Below is the commented code and its snapshot and video demonstration.
Processing Code: Ultrasonic Distance Measurement
import processing.serial.*;
Serial myPort; // Create object from Serial class
String Sensor_data=""; //Initialization the string for the Input sensor data
void setup () {
size (600,350);
//create window with 600x350
myPort = new Serial(this, "COM3", 9600); //Connect myPort with COM3
//where arduino is actually connected
myPort.bufferUntil('\n');
}
void draw (){
background (#ac0000); // (RED)
//Set Background color
fill(0); //Fill Black colour for the rectangle
rect(0, 0, 600, 100); //Draw the rectangle (X position, Y position, X size, Y size)
fill(#FFFFFF); //Text color (white)
textSize(30); //text size
text("Ultrasonic Distance Measurement",60,70);
// 50:X direction Movement, 70:Y direction movement of text
// To write the title, the position of the Title
textSize(30); //text size of Sensor Data
text(Sensor_data,300,200); //280 is X coordinate, 200 is Y coordinate
textSize(30); //text size of Distance in cm
fill(#FFEF00); //Colour fill for the text Distance (White)
text("Distance : cm",150,200); //Type the text
fill(0); //Fill Black colour for the rectangle
rect(0, 280, 600, 70); //Draw the rectangle (X position, Y position, X size, Y size)
}
void serialEvent (Serial myPort) {
Sensor_data=myPort.readStringUntil('\n'); //Reads incoming data string
}
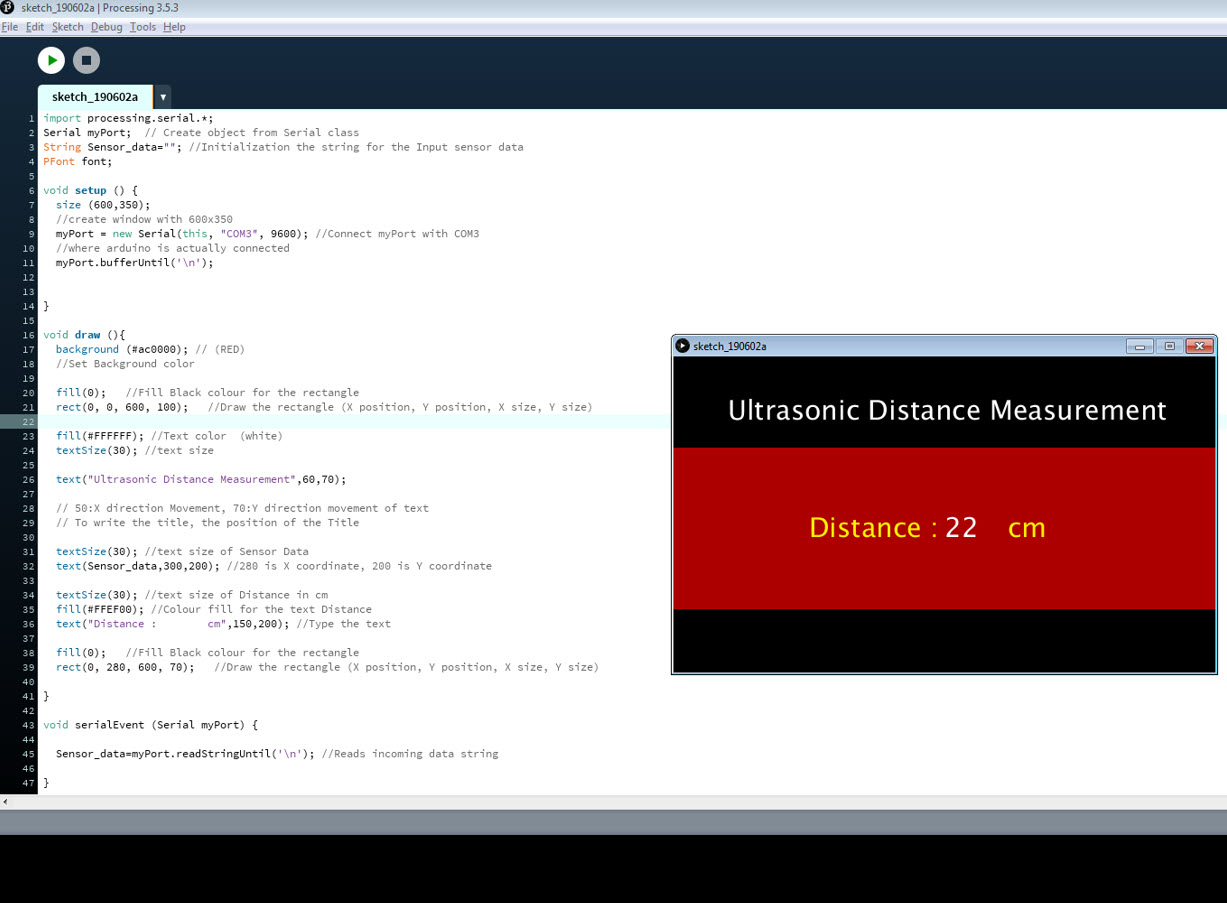
Overall I was able to get the codes work smoothly, No issue was faced during the process of Interface and Application Programming./p>
Resources Utilized
- I utilized these resources for this task:
- Processing
- Arduino IDE